CS 149 Intro to Programming
Fall 2020
CheckStyle Lab -- Creating Stylish Code
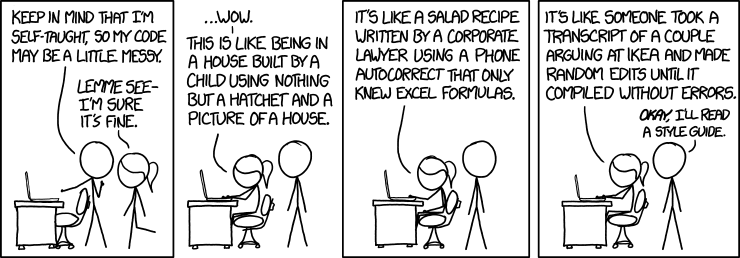
Learning Objectives
- List (and appreciate) the reasons that writing code in the proper style is important
- Install, configure, and use Checkstyle to validate the format of your Java code
- Start using folders to organize the code you will develop this semester
- Define and follow the checkstyle rules for comments,Javadoc, variable names, constants, literals, indention, and whitespace.
Actions/Deliverables
- Download the required Checkstyle files and configure jGRASP to use them (the checkstyle icons should appear)
- Correct all style errors in Payroll.java and submit your assignment to Autolab (collaboration allowed, but all must submit).
Background
- Code Conventions (April 1999)
- Google Java Style (March 2014)
In this course, you will follow a simplified version of these guidelines. Rather than memorize a long list of rules, you should develop good habits and intuition when it comes to style.
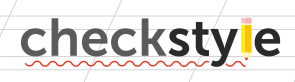
Checkstyle is a tool (see checkstyle.sourceforge.net) that validate that your code follows a set of style guidelines.
Part 1: Configure jGrasp to run Checkstyle
Download the following files and place them somewhere that is not specific to this lab (as you will need them for all future work). A suggestion would be to create a folder/directory specifically for checkstyle (like ..../CS149_Fall2020/checkstyle).
- checkstyle-8.35-all.jar
- cs149.xml (you will need to right click on this file and select save as to download it)
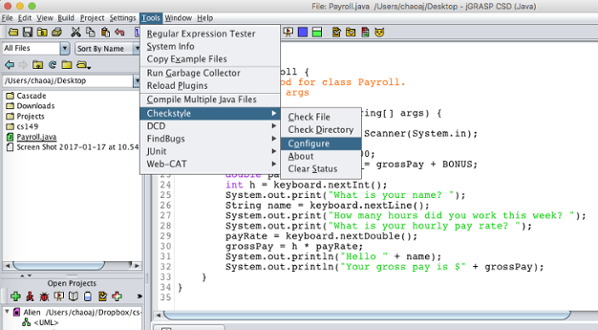
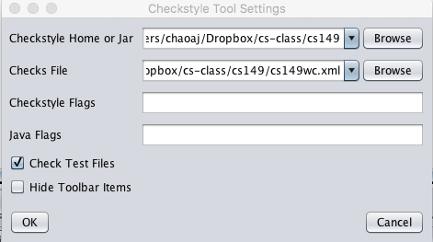
- Set Checkstyle Home to the directory/folder where you downloaded the checkstyle-8.35.-all.jar file.
- Set Checks File to the full path/location of the cs149.xml file you downloaded.
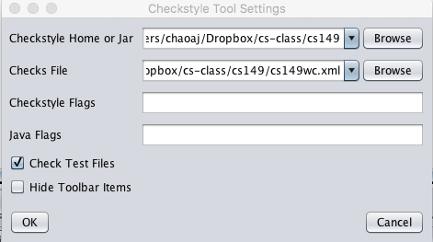


Configure jGRASP to Promote Good Style
- Check the box for soft tabs (this inserts spaces instead of the special tab (\t) character
- Set the tab size to 3 (this is the default, so, you should just need to verify this).
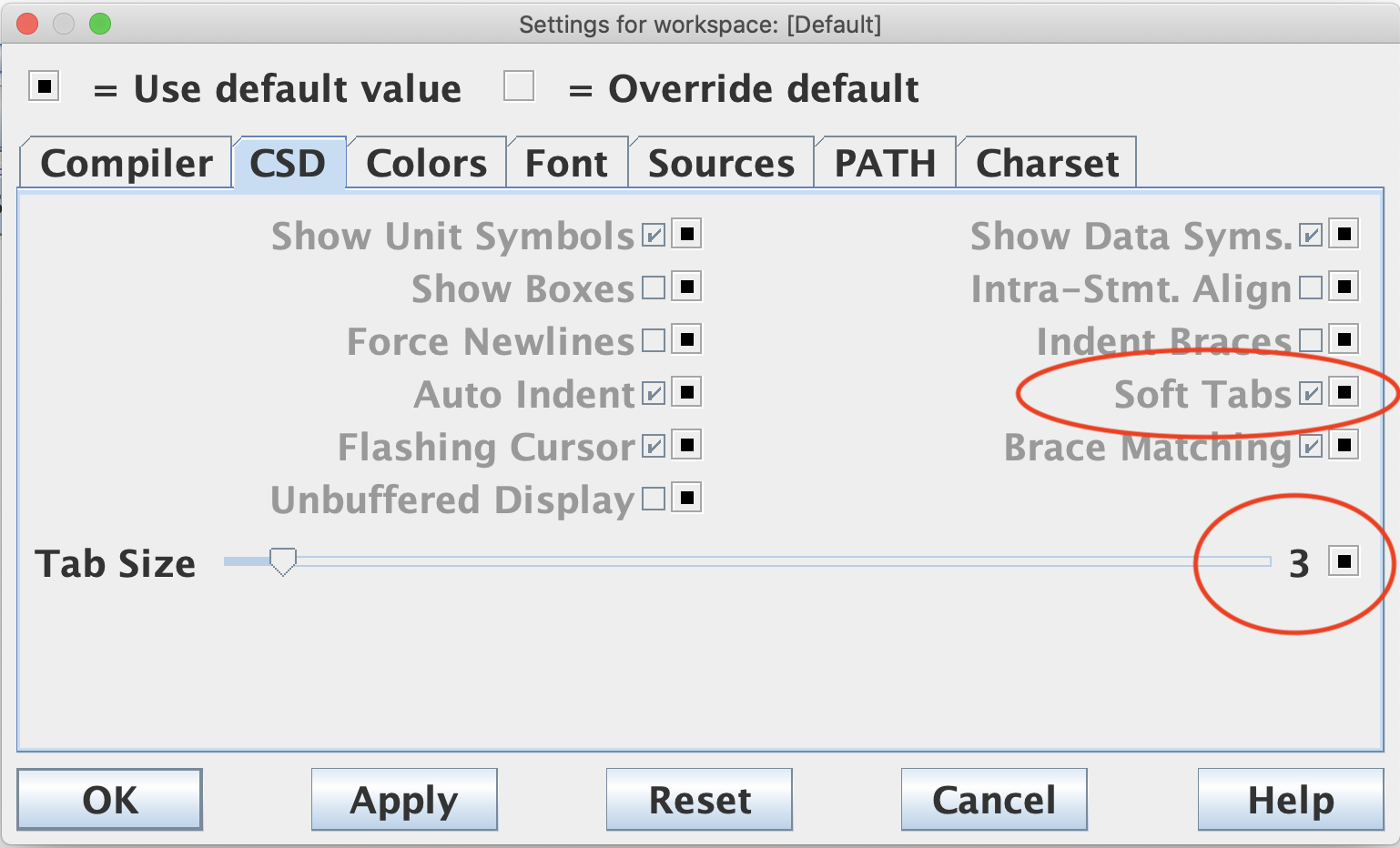
Part 2: Styling a program: An example
Lab instructions
Actions:
- Directory Structure If you haven't already, you should create a top-level CS149 folder/directory to organize all your labs and other assignments for the semester. Create a directory called CheckstyleLab under your CS149 folder.
- Download Payroll.java and move Payroll.java into the Checkstyle directory.
- Correct all of the style errors in this file, using
the (
) icon to run checkstyle.
- After eliminating all of the style errors, submit your assignment to Autolab. Each student must submit a copy to Autolab to receive credit.
Checkstyle Rules
Style Part A: Comments
- Every class must contain a Javadoc comment (below
is an example). This comment contains:
- The first line must be a //* as shown in the example. The other lines must start with the aligned astericks characters.
- A short description of what the class does. This description must end with a period (otherwise Checkstyle will mark it as an error).
- The next line starts with @author, followed by the name of the original person who wrote the class
- The next line starts with @version. In this class, you can just put the due date of the assignment for the version.
- The honor code acknowledge is next.
- This is a block comment, which ends with the sequence */. Java treats all information between the //* and the */ as a comment (which can span many lines, as it does in this example).
/** * Overall description of the class goes here. * * @author Your name goes here * @version Due date goes here * Acknowledgements: I received no outside help on this assignment and have * abided by the JMU Honor Code. */
- All methods (including main) must contain an applicable Javadoc comment as shown in the following example.
- Inline comments (//) should describe major structures and steps within a method.
- All comments should use normal English spelling and grammar. Phrases are OK
- Comments must come before the code that they are describing or on the same line.
/**
* Overall description of the method goes here.
*
* @param parameterName describe the first parameter
* @param parameter2 description of 2nd parameter
* @return
*/
Style Part B: Names
- All names should be descriptive and readable.
(
subTotal
rather thans
,grade
rather thangrd
) - Multiple-word names should use capital letters to separate words.
(
subTotal
, notsub_total
) - Variable and method names should begin with a lowercase letter, and:
- Variable names should be nouns or noun phrases.
(
studentName
orsubTotal
) - Method names should be verbs or verb phrases.
(
printLine
oraddColumn
)
- Variable names should be nouns or noun phrases.
(
- Class names should begin with a capital letter and use title case.
(
HelloWorld
) - Constant names should be all caps with an underscore separator.
(
PI
orINTEREST_RATE
)
Style Part C: Declarations
final double CENTIMETERS_PER_INCH = 2.54;
centimeters = inches * CENTIMETERS_PER_INCH; // NOT inches * 2.54;
- All constants should be named and initialized at the top of the method in which they are used.
- All variables should also be declared at the top of the method, directly after any constant declaraions.
- It is strongly recommended (in CS 149) to separate variable declaration and initialization statements.
- Each declaration should be on its own line. Comment to the right if the name is not self-explanatory.
Scanner input = new Scanner(System.in); // discouraged
Scanner input;
input = new Scanner(System.in);
Style Part D: Literals
- Numeric literals should be of the correct type for the context in which they are used.
// integer expressions should use integer literals
int a;
double b;
a = 2;
b = 2.0;
// double expressions should use double literals
double x;
double y;
double average;
average = (x + y) / 2.0; // NOT 2, which is an integer
Style Part E: Indentation
- Subsections of code should be indented consistently with three spaces.
- Always use three space characters, not tab characters, for indentation.
- Statements too long for one line should be indented on subsequent lines.
- All blocks of code (even if one line) should be surrounded by curly braces.
- Left braces must appear on the same line as the structure header.
Style Part F: Whitespace
- There should be a space after cast operators, commas, and //'s.
- Use whitespace to separate logical segments of code. There should be a blank line after variable declarations.
- Lines should be kept to a short length (< 80 chars). You should be able to see the full line in your text editor.
- Binary operators should be separated from their operands by a single space. (
sum = myGrade + yourGrade;
) - One exception is the dot (
.
) operator, which should not have space surrounding it. (System.out.println();
) - Unary operators should not be separated by a space. (
myGrade++;
)
This lab is a modified version of the one developed by Professor Alvin Chao.