Homework 1: Basic Python
Objectives
The goal of this homework is to verify mastery of the basic features of the Python programming language including functions, variables, loops, strings, and lists.
This lab is based on work done in previous labs: shapes and strings.
Part 1: Checkerboard
Building on the code that you wrote in the first lab, write a new turtle graphics function with the following signature:
checkerboard(x, y, rows, columns, size)
This function should create a rows x columns
grid of colored
squares. The lower-left corner of the checkerboard will be at position (x,y).
The size
parameter indicates the width of the individual squares.
Alternating squares should be filled with blue in a checkerboard pattern,
and the lower-left square should be filled in. You will need to look through
the documentation
for the begin_fill()
and end_fill()
functions. Here
is an example of what a 3x8 checkerboard should look like:
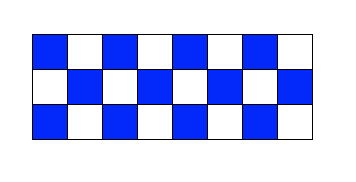
Part 2: Polygon File Drawing
Write a function with the following signature:
draw_polygon_file(fname)
This function should take a file name as a parameter, and draw the polygons
stored in that file. You should re-use your polygon
function from
the shapes lab. The file format consists of a series of polygon specifications
with four integers per polygon and one polygon per line. The line format for one
polygon is:
x_pos y_pos sides size
Each integer is separated by a space. Here is an example of two lines from such a file:
0 0 3 50 50 100 4 20
The above lines would encode a 50-pixel wide triangle at position (0, 0), and a 20-pixel wide square at position (50, 100).
You will need to use some very basic Python file I/O for this part, and for
this you should reference Section 1.6 in your textbook as well as the
documentation. I recommend using the readlines()
function to
read all lines into a list after you have open()
'ed the file. That
will simplify the process of splitting the strings and using the parameters to
draw polygons.
Here are some sample files you can test with:
Part 3: String Formatting
Write a function with the following signature:
format_str(description, values)
This function should return a reformatted version of
description
, where special identifier words like "$3
"
have been replaced by the specified element from values
. The
special identifiers should always be a single word (no spaces) composed of a
single dollar sign ("$") followed by the zero-based index of the desired value.
Your function should work regardless of whether the values are strings,
integers, or floating-point numbers. Your function should also check to make
sure the given index is within the bounds of the given list. If it is not, your
function should insert the text "ERROR
".
Here are some examples:
format_str("Hello $0", ["World"]) # result: "Hello World" format_str("The sum of $0 and $1 is $2", [3,5,8]) # result: "The sum of 3 and 5 is 8" format_str("The name is $1 -- $0 $1", ["James", "Bond"]) # result: "The name is Bond -- James Bond" format_str("Names: $0 $1 $2 $3", ["Mike", "Nathan"]) # result: "Names: Mike Nathan ERROR ERROR"
To extract the index number from a special identifier, you may wish to use a technique called slicing. You can find information about it in the Python string documentation.
Submission
DUE: Friday, September 5, 14:30 (2:30pm) ET
You must submit a single file named hw1.py
with all of your
code on Canvas. Open Canvas, go to the "Assignments" page, open "Homework 1" and
click "Submit Assignment" (on the right side). Upload a singled, unzipped
source file.
Make sure that you document your code appropriately, including docstrings for all functions and regular comments where appropriate. Include a docstring at the top of the file with the homework title, the date, your name, and the following statement:
This submission compiles with the JMU Honor Code. All code was written by the submitter, and no unauthorized assistance was used while completing this assignment.
Also, check your code thoroughly to make sure that it complies with the class style guide.
NOTE: While this homework consists of programming problems, it is not categorized as a programming assignment in this course, and therefore the 72-hour late policy does NOT apply. No late submissions will be accepted on this homework.
NOTE: You may re-use code from a previous lab, even if you wrote it in a group with others. If you did work in a group for the lab, please acknowledge this in the comments at the top of your code. All new code written for this homework must be your own work; do not share it with others.