Shapes Lab

Objectives
The goal of this lab is to get comfortable working with basic features of the Python programming language including the Python interpreter, variables, user defined functions and loops.
This lab is based on an in-class activity originally written by Nathan Sprague.
Introduction
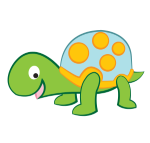
Logo is a popular educational programming language that uses turtle graphics to
demonstrate programming concepts. Python contains a module called
turtle
that implements similar features. In this lab we will be
using this module to explore basic Python programming.
The following example illustrates the process of importing and executing
turtle
commands:
Python 3.4.0 (default, Apr 11 2014, 13:05:11) [GCC 4.8.2] on linux Type "help", "copyright", "credits" or "license" for more information. >>> from turtle import * >>> forward(100) >>> left(90) >>> forward(100)
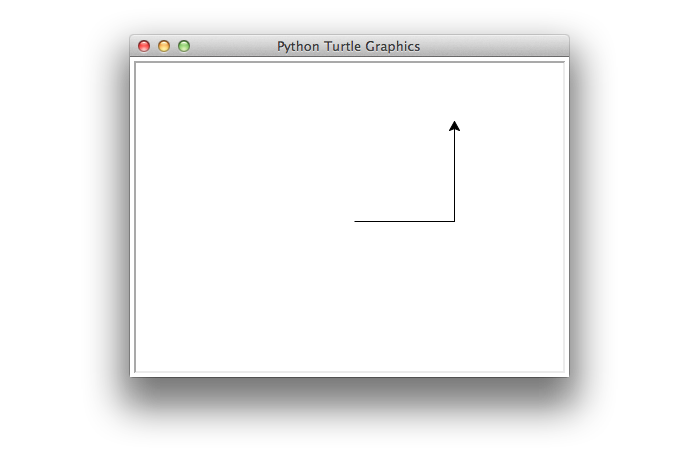
Complete documentation for the turtle module can be found in the Python standard library documentation. The following functions should be enough to complete this lab activity:
forward(distance) |
Move the turtle forward/backward the indicated distance (in pixels). |
left(angle) |
Turn the turtle left/right by the indicated angle (in degrees). |
penup() |
After penup() is called, the turtle will not draw
until pendown() is called. |
goto(x, y) |
Move the turtle to the indicated position, where the origin (0,0) is the center of the screen. The orientation is not changed. |
setheading(angle) |
Set the orientation of the turtle to the indicated angle. Zero degrees points to the right, and ninety degrees points up. |
exitonclick() |
This command prevents the turtle screen from closing until it is clicked by the user. This should be the last command in your main code. |
Note that you must be using a version of Python that has Tk support, because the turtle
module uses the
tkinter
module for graphics output. Both labs in ISAT (248 and
250) should have this software installed.
Exercises
- Getting Started
- If you haven't already, open a terminal window and start the Python
interpreter by typing "
python3
" at the prompt. - Spend a few minutes experimenting with the
turtle
module commands from the table above.
- If you haven't already, open a terminal window and start the Python
interpreter by typing "
- Rectangles
- Save the file shapes.py to your desktop (or somewhere else that is easily accessible).
- Start Idle3 (or the editor of your choice) and open
shapes.py
. - Take note of the
import
statement at the top of the file. We will learn about this statement later, but for now you should only know that it allows you to callturtle
module functions without prefacing them with the module name. In other words, you may simply write "forward(100)
" instead of "turtle.forward(100)
". In general, this is a bad idea (for reasons we will discuss later), but for the purposes of this initial lab activity we use it to simplify things and produce cleaner code. - Add a new function with the following signature:
rectangle(x, y, width, height)
This function should draw a rectangle with the indicated width and height. The lower-left corner of the rectangle should be at position (x, y). - Modify the main function so that it calls your newly created
function. Test your program in IDLE by pressing F5 or clicking on "Run
Module" under the Run menu. You can also execute your code by typing
"
python3 shapes.py
" in a command-line terminal prompt after you have changed to the appropriate folder. Try out both methods.
- Polygons
- Add a new function with the following signature:
polygon(x, y, sides, size)
This function should draw a polygon with the indicated number of sides at position (x, y). The length of each side is determined by thesize
parameter. This function should work for any value ofsides
that is greater than 2. Do not hard-code the function for any particular number of sides.
Hints:- This could be accomplished using either a
for
loop or awhile
loop. The best approach is to use afor
loop along with therange
function to control the number of loop iterations. - Notice that the turning angles required to draw a square were all 90 degrees and that 90 = 360.0 / 4.
- This could be accomplished using either a
- Update the main function so that it includes several calls to your
newly defined
polygon
function. - If you have extra time or would like more practice later, try
writing some code that will draw a series of polygons with the same
general size but with an increasing number of sides (as in the graphic
at the top of this page). You will need to change the side length of
the polygons as you increase the number of sides. Here are some
questions to think about:
- Do you need to increase or decrease the side length as you increase the number of sides?
- After how many sides is the polygon indistinguishable from a circle?
- Does this number vary if you change the initial size?
- Add a new function with the following signature:
- Rows
- Add a new function with the following signature:
row(x, y, count, size)
This function should draw a horizontal row of squares with the lower-left corner of the row at position (x, y). Thecount
andsize
parameters indicate the number of squares in the row and the size of each square respectively. Your function should leave a small (5-10 pixel) fixed space between each square.
Do not copy/paste code from the existingsquare
function! Yourrow
function should invokesquare
with appropriate parameter values. - Update the main function so that it includes several calls to your
newly defined
row
function.
- Add a new function with the following signature:
- Grids (optional)
- Write a new function with the following signature:
grid(x, y, rows, columns, size)
This function should create arows x columns
grid of squares. The lower-left corner of the grid will be at position (x,y). Thesize
parameter indicates the width of the individual squares
For further experimentation, make alternating squares filled in a checkerboard pattern. You will need to look through the documentation for thebegin_fill()
andend_fill()
functions. Here is an example of what it could look like: - Update the main function so that it includes several calls to your
newly defined
row
function.
- Write a new function with the following signature:
- Sierpinski (optional)
- If you want some practice with a more complex algorithm, try
writing a function that draws a Sierpinski
triangle. You should be able to reuse the
polygon
function you wrote earlier, and you may wish to use thespeed()
ortracer()
/update()
functions to speed up your code development. Here is an example of what the end result should look like:
Hint: use recursion!
- If you want some practice with a more complex algorithm, try
writing a function that draws a Sierpinski
triangle. You should be able to reuse the
Submission
This lab will not be graded so there is nothing to submit. Make sure you keep a copy of your code for future reference; you may also need it for a homework assignment. If you would like to discuss your solution or any problems you encounter while working on this lab, please come to office hours or post on Piazza.