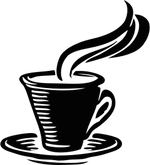
PA6 - Decaf x86 Code Generation
Goal
The goal of our semester-long project is to gain experience in compiler implementation by constructing a simple compiler. We will be implementing a compiler for a variant of the well-known Decaf language, which is a simple Java-like procedural programming language.
The goal of this part of the project is to generate x86 assembly code for Decaf programs.
Description
As before, you will need to re-download or modify the main driver. There are no modifications to DecafAST.py for this assignment.
You should augment your DecafCodeGen.py
file with a new
generate_x86
function. It should take a TACProgram
and
return a string containing x86 assembly. The resulting assembly should adhere to
standard x86 cdecl calling conventions and should compile on the server using
"gcc -O0".
Sample Input
class Program { void foo() { bar(); } void bar() { } void main() { foo(); bar(); } }
Sample Output
.section .bss .section .data .section .text .globl main foo: push %rbp mov %rsp, %rbp call bar leave ret bar: push %rbp mov %rsp, %rbp leave ret main: push %rbp mov %rsp, %rbp call foo call bar leave ret
Submission
DUE: Friday, Apr 17, 2015, at 23:59 (11:59PM) EST
Recommended file names (for Python):
Decaf.g4 (grammar, previous project) DecafLexer.py (auto-generated using ANTLR4) DecafParser.py " DecafListener.py " DecafCompiler.py (driver, provided above) DecafAST.py (AST framework, provided above) DecafASTConverter.py (converter, previous project) DecafAnalysis.py (analysis routines, previous project) DecafCodeGen.py (TAC/x86 generation routines)
Submit a zip or tarball with your complete solution on Canvas. Your code must compile and work on the class server. Part of your grade will be based on automated testing and part will be based on style considerations. Please make sure that your project adheres to the specification exactly to avoid difficulties with the automated testing framework, and make sure that your code is clean and documented.