Warm-up Quiz Questions
03 C Intro
Consider the following C program:
#includevoid do_stuff (int a, int *b) { int triple = a * 3; b = &triple; } int main () { int n = 0; do_stuff(5, &n); printf("%d", n); return 0; }
What will happen if we run this program?
04 C arrays and strings
Which of the following expressions will evaluate to true if and only if a single command-line parameter is passed to "./intro" for P0?
- argc == 0
- argc == 1
- argc == 2
- argc != NULL
- There is not enough information to know.
Which of the following expressions will evaluate to true if and only if the first command-line parameter passed to "./intro" for P0 starts with "-g"?
- strncmp(argv[0], "-g", 2) != NULL
- strncmp(argv[0], "-g", 2) == 0
- strncmp(argv[0], "-g", 1) == 0
- strncmp(argv[1], "-g", 2) != NULL
- strncmp(argv[1], "-g", 2) == 0
- strncmp(argv[1], "-g", 1) == 0
- None of these will work.
05 C structs and I/O
Consider the following C code:
typedef union { short tiny; int medium; long big; } thing_t;
Assume that the 'short' type must be at least two bytes, the 'int' type must be at least four bytes, and the 'long' type must be at least eight bytes. What is the minimum amount of space required to store a 'thing_t' variable?
- 8 bytes
- 14 bytes
- 16 bytes
- 24 bytes
- There is not enough information to know.
06 C Miscellaneous
What's wrong with the following C code, assuming that we compile with --std=c99 and the 'nodelist_t' struct type has an integer element called 'size'?
bool nodelist_is_empty(nodelist_t* list) { if (list->size == 0) { return true; } else { return false; } }
- It won't compile due to a syntax error
- It's not valid according to the C99 standard
- It invokes undefined behavior
- It's silly!
- Nothing -- this code is totally fine
07 Binary Information
Which of the following C expressions perform exactly two pointer dereferences, assuming p is a pointer variable? (Select all that are correct.)
- *p
- p[0]
- *p[0]
- p[0][0]
- **p[0]
- None of these
08 Integers
What is the decimal value of an int8_t variable in C if the actual bits being stored are equivalent to 0x80 in hex?
- -128
- 16
- 64
- 128
- Not enough information to determine
09 Binary Arithmetic
Consider the following binary subtraction problem. Assuming 4-bit representations, is this an overflow in unsigned, two's complement, both, or neither? (note the borrow into the most-significant bit!)
0011 - 1001 ====== 1010
- Overflow in unsigned ONLY
- Overflow in two's complement ONLY
- Overflow in BOTH unsigned and two's complement
- Overflow in NEITHER unsigned nor two's complement
- Not enough information to determine
BONUS: What about 0011 - 0101?
Notes:
2 0011 3 3 - 1001 - 9 - -7 ====== ==== ==== 1010 10 -6 !!!! !!!! 12 0011 3 3 - 0101 - 5 - 5 ====== ==== ==== 1110 14 -2 !!!! (ok)
10 Floating Point
What is the decimal value encoded as 0x44 with a four-bit exponent and a three-bit significand? (i.e., 0 1000 100 in binary)
HINT: the bias for a four-bit exponent is 7.
- 0.2
- 1.1
- 3.0
- 8.0
- Not enough information to determine
BONUS: Encode the decimal value 0.125 in the same format.
11 Assembly Intro
What is the return value (i.e., the value of the RAX register) at the end of the following x86-64 assembly program?
.globl main main: mov $2, %rax mov $3, %rbx mov $7, %rcx imul %rbx, %rcx imul %rcx, %rax ret
- 6
- 7
- 14
- 21
- 42
- None of the above -- the program will crash
12 Assembly Data Movement
The following is a hex dump of a program's memory starting at address 0x400 (in little endian ordering).
0400 00 00 00 00 00 00 00 00 01 02 03 04 05 06 07 08 0410 15 23 ae 7c 01 02 59 68 aa bb cc dd 34 56 78 90
What is the decimal value of the integer number stored at address 0x408? (You may need to use a calculator.)
- 0
- 1
- 513
- 67,305,985
- 578,437,695,752,307,201
- Not enough information to determine
13 Assembly Control Flow
What is the appropriate C-expression for CONDITION if when given the following C code:
void cond(long a, long *p) { if (CONDITION) { *p = a; } }
GCC generates the following assembly code:
# void cond(long a, long *p) # a in %rdi, p in %rsi cond: testq %rsi, %rsi jz .L1 cmpq %rdi, (%rsi) jge .L1 movq %rdi, (%rsi) .L1: ret
- p || a < *p
- p || a > *p
- p && a > *p
- p && a >= *p
- p && a < *p
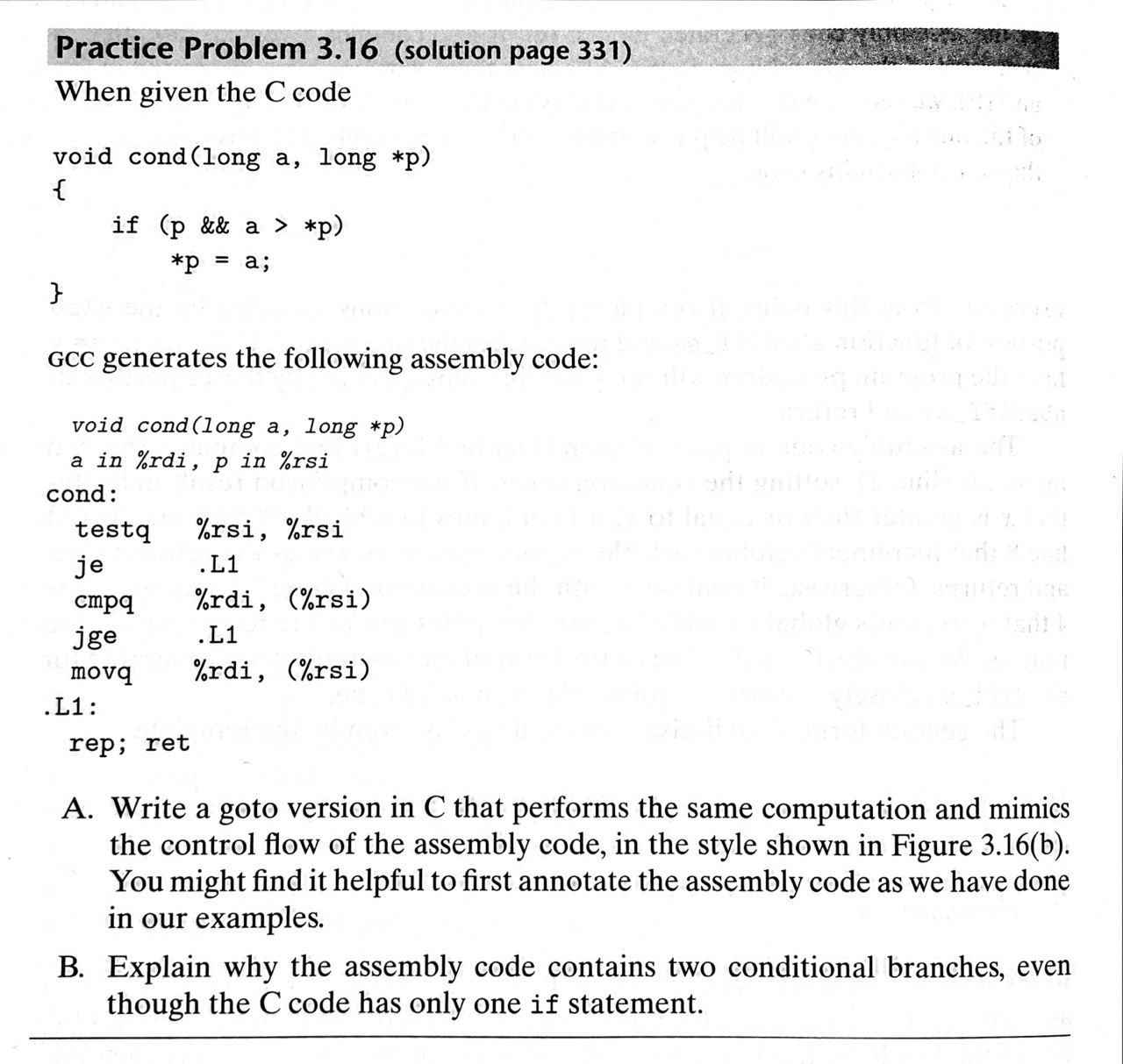
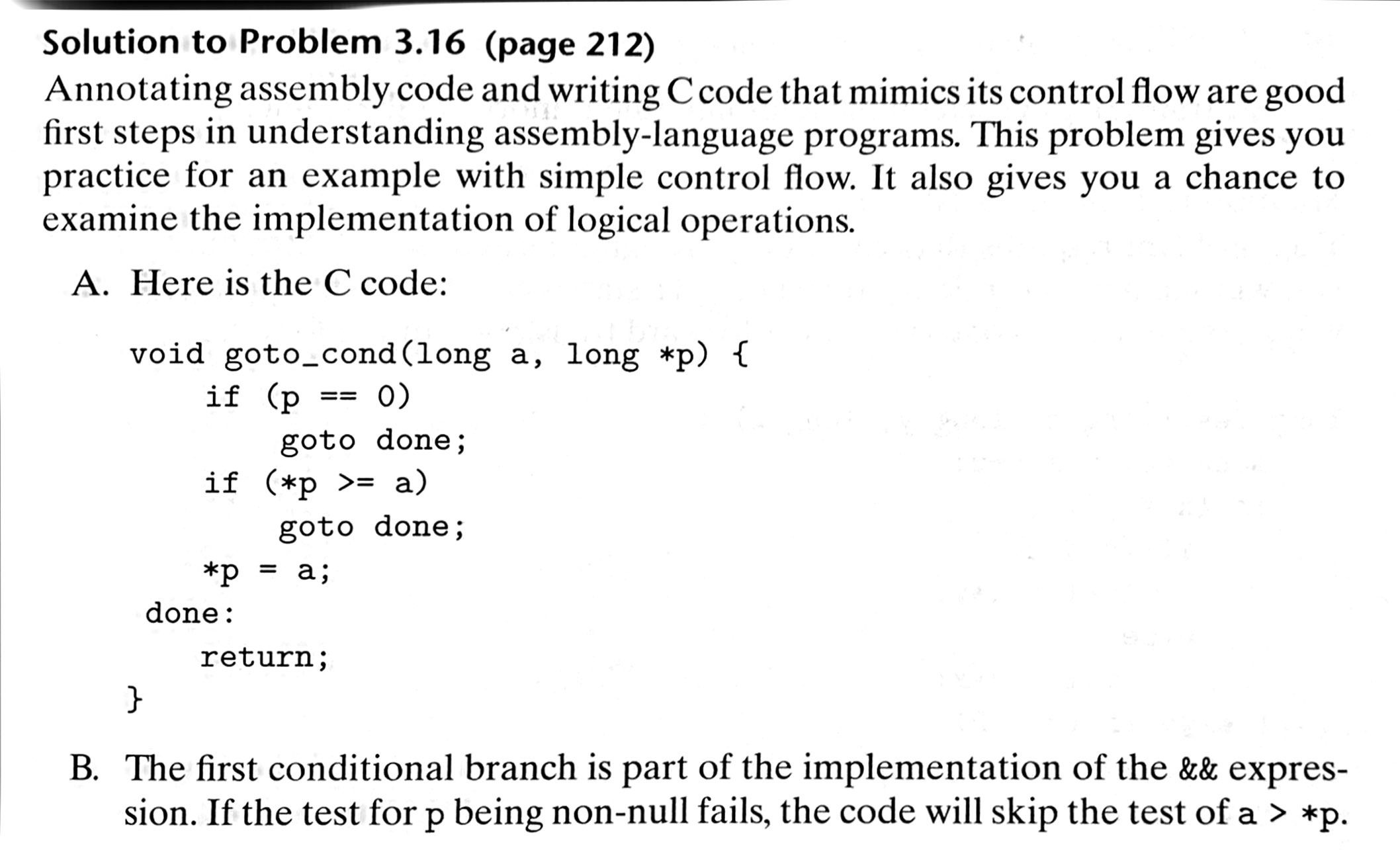
14 Assembly Procedures
What value will be on the top of the stack after the following callq instruction executes?
0x4004f7 callq 4004db 0x4004fc halt
- 0x4004db
- 0x4004f7
- 0x4004fc
- Some other value.
15 Assembly Miscellaneous
Assume an array of 32-bit integers is stored in memory starting at address 0x600240. What is the address of the first byte of the fourth element of the array?
Hint: draw a diagram!
- 0x600243
- 0x60024c
- 0x600250
- 0x600252
- 0x600256
16 Y86 Intro
What is the instruction code (icode) and the instruction function (ifun) of the following Y86 instruction?
0x114: 60 10 | addq %rcx, %rax
Hint from the textbook: "Every instruction has an initial byte identifying the instruction type. This byte is split into two 4-bit parts: the high-order, or code part, and the low-order, or function part."
- icode: 0, ifun: 6
- icode: 1, ifun: 0
- icode: 6, ifun: 0
- icode: 6, ifun: 1
- icode: 60, ifun: 10
17 Combinational Circuits
What is the result of x NAND x?
- 0
- 1
- x
- !x
- Not enough information to answer
18 Sequential Circuits
The following is an AND/OR SR latch and its truth table. What will happen when the "S" input is turned on, assuming both the "R" input and the previous value for "Q" are both off?
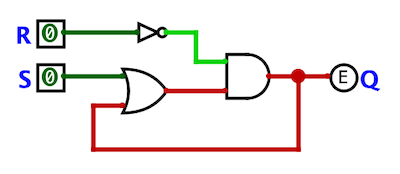
R | S | Q (before) | Q (after) |
---|---|---|---|
0 | 0 | 0 | 0 |
0 | 0 | 1 | 1 |
0 | 1 | 0 | 1 |
0 | 1 | 1 | 1 |
1 | 0 | 0 | 0 |
1 | 0 | 1 | 0 |
1 | 1 | 0 | 0 |
1 | 1 | 1 | 0 |
- Q will turn on
- Q will turn off
- R will turn on
- S will turn off
- The circuit will explode
- Not enough information to answer
19 CPU Architecture
How does the clock period (cycle length) of a CPU typically change when converted from a sequential CPU design to an equivalent but pipelined CPU design?
HINT: Consider this slide, added at the end of today's slide deck after the original CS 261 videos were recorded:
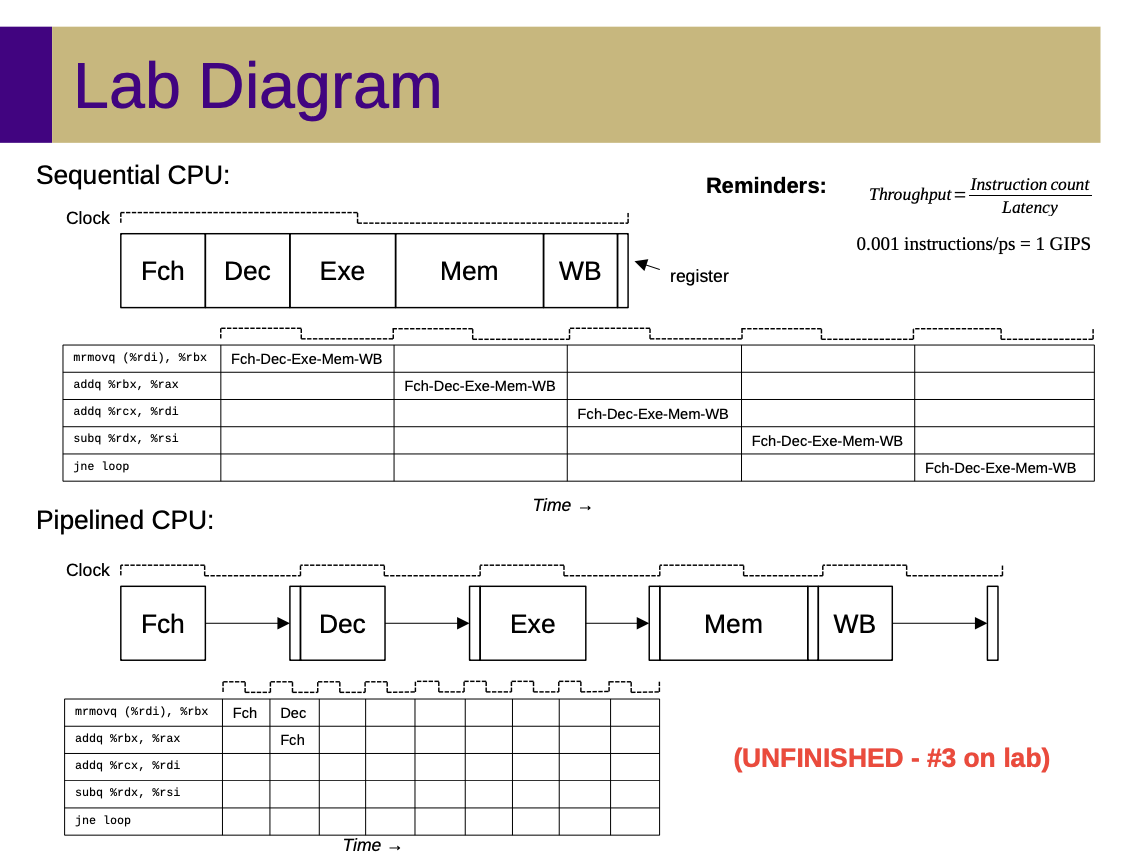
- The clock period will typically increase
- The clock period will typically stay the same
- The clock period will typically decrease
- The result depends on the number of instructions being executed
- Not enough information to answer
20 Y86 Semantics
What is the minimal number of bits required to provide enough unique register IDs for 32 registers?
HINT: How many bits are required in Y86?
- 4
- 8
- 16
- 32
- 64
- None of these are correct
21 Memory
Suppose that the last three memory addresses accessed by a program were 0x510, 0x514, and 0x518, in that order. Which of the following addresses is the most likely to be accessed next?
- 0x510
- 0x519
- 0x51c
- 0x520
- 0x522
- None of these are correct
22 Caching
Assume the following cache for an 8-bit address: s=3 bits for the set index (S=8 sets), E=1 (direct- mapped), and b=2 bits for the byte offset within a line (B=4 bytes). This leaves t=8-3-2=3 bits for the tag.
What is the set number for address 226 (0xE2)?
- 0
- 2
- 3
- 7
- 14
- None of these are correct
23 Virtual Memory
How does the addition of virtual memory to a system affect memory access times?
- They will increase.
- They will stay roughly the same.
- They will decrease.
- It depends on the system.
24 Exceptions and Processes
What will be the output of running the following program?
int main () { if (fork() == 0) { printf("jmu\n"); } else { printf("dukes\n"); } return 0; }
-
jmu
-
dukes
-
jmu dukes
-
dukes jmu
- The answer cannot be determined.
25 Files
Assume a C program opens "story.txt". After that, the process forks and the child opens "letter.txt". How many open file descriptors will there now be in the parent process?
Follow-up question: how about in the child process?
- 1
- 2
- 3
- 4
- 5
- None of these are correct.
26 Threads
Which of the following is the best description of a data race (also called a race condition), as defined in this class?
- More than two threads access the same location in static memory.
- More than two threads access the same location in heap memory prior to the memory being freed.
- Correct output depends on a program's instructions executing in a particular order across multiple threads.
- A program runs slower when run with more than a single thread.
- None of these are correct.