Homework 1
Introduction
The goal of this homework is to solidify your understanding of the C language and your ability to write simple programs in C. You will be finishing up some of the previous labs and submitting your solutions for grading.
For this homework, you must adhere to the specifications below, NOT the original lab pages. Please read this page carefully. We will attempt to highlight any differences from the exercises as they appeared on the labs, but you are responsible for making sure that your code implements this homework assignment correctly.
Starter Files
You should put your code for this homework into a file named hw1.c
, that should compile against our hw1.h. If you wish, you may start from our boilerplate files:
The boilerplate archives include a main.c
(for testing your graphical functions) and a testsuite.c
(for testing your other functions). You will only need to submit hw1.c
.
Lab 2: Grids
Write a function with the following signature:
void draw_grid(int x, int y, int rows, int columns, int size)
IMPORTANT: In Lab 2, this function was called "grid
", but now it should be called "draw_grid
".
This function should create a rows x columns
grid of squares each of size size
with the lower-left corner at (x, y)
. It is important to note that the size
parameter indicates the width of the individual squares, not the size of the overall grid.
Alternating squares should be filled in a checkerboard pattern with the color blue (RGB values: 0, 0, 255). You will need to look through the documentation for the turtle_begin_fill()
and turtle_end_fill()
functions.
The output from the sample main.c
should look something like this:
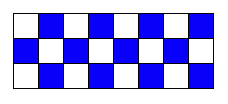
A grid of alternating color blocks
You may assume that rows
, columns
, and size
are all positive integers.
HINT: It is not required for this homework, but you will probably find it very helpful to first write a draw_square()
function like the following:
void draw_square(int x, int y, int size)
This function should draw a square of size size
with the lower-left corner at (x, y)
.
Lab 3: Finding the second minimum value
Create a function that returns the 2nd minimum value of an array. Here is the function signature:
int second_min(int nums[], int nums_len)
The input to this function is the array of integers and the length of the array. If there are duplicate elements, you should only count them once. You may assume that the input array has at least two elements.
HINT: It is not required for this homework, but you may find it helpful to first write a min()
function like the following:
int min(int nums[], int nums_len)
This should take as input an array of integers called nums
. The second parameter, nums_len
is the length of the array. After this function is working, think about how you would extend it to work for the second minimum.
HINT: You may wish to use the INT_MIN
or INT_MAX
constants for this exercise. They are defined in the limits.h
standard header file.
Lab 3: Palindromes
Write a routine with the following signature:
bool is_palindrome(char word[])
Your function should determine if the given string is a palindrome word (can be written the same way forwards and backwards - like "madam", "otto", "anna", "redivider", "kayak", and "racecar". This function should do a strict character-based test; i.e., it should not ignore punctuation and whitespace. Empty words and one-character words should be considered palindromes for the purposes of this function.
Lab 3: Same things in strings
Write a routine with the following signature:
void same_things(char string1[], char string2[], char common[])
This function should search through the two given strings and determine the longest sequence of characters that match at any point in the string, saving the longest match to the string common
.
IMPORTANT: In Lab 3, this function just returned the first index of the longest match; now it should save all the characters of the match to the common
character array.
For example, given the following code:
char str1[] = "This is a long sentence of some sort.";
char str2[] = "This is not a long sentence of some sort";
char dest[1024];
same_things(str1, str2, dest);
After that code executes, dest
should contain the following string:
" a long sentence of some sort"
Note the leading space in the output above. Also, don't forget to add the null terminator character ('\0'
) to the output character string!
You may assume that common
is large enough to hold the longest common character sequence.
Lab 4: Drawing icons
Define a function called draw_icons
with the following signature:
void draw_icons(icon_t icons[], int num_icons, int start_x, int start_y)
This function takes as input an array of icon_t
structs (defined in hw1.h
and the number of icons in the array as well as a starting (x, y) position. The function should draw them in a row (according to their icon_type
, also defined in hw1.h
) using the turtle graphics library.
The output from the sample main.c
should look something like this:
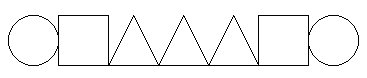
A row of seven icons
Submission
DUE DATE: Friday, September 18, 2015 at 23:59 EDT (11:59pm)
Submit ONLY your completed hw1.c
file on Canvas using the appropriate assignment submission. Make sure the file contains a comment field at the top with your name and a statement of compliance with the JMU honor code.
Please check your code carefully and make sure that it complies with the project specification. In addition, make sure that your code adheres to general good coding style guidelines. Fix any spelling mistakes, incorrect code formatting, and inconsistent whitespace before submitting. Make sure you include any appropriate documentation.