Exception Lab
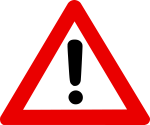
Objectives
The goal of today's activity is to practice working with exceptions and exception handling in Python.
Part 1: Tracing Exceptions
The file word_writer.py contains a simple command-line utility that creates a file containing any number of repetitions of a single word. Here is the docstring describing the script:
Command line utility for creating a file that contains many copies of a single word. Usage: word_writer.py FILE_NAME WORD COUNT Example: $ python3 word_writer.py hellos.txt hello 3 This would result in the creation of a file named 'hellos.txt' with the contents: hello hello hello This utility will do nothing if the file already exists.
Download the file and read it over to get a feel for how it works. DON'T EXECUTE IT YET.
Once you have read the file, trace its execution for each of the invocations below. For each one, write exactly what will be printed to the terminal on a separate sheet of paper. Once you are satisfied with your prediction, go ahead and run the script with the indicated arguments.
Keep in mind that exception handling in Python (as in Java) respects the
exception class hierarchy. For example, the following exception block will be
triggered if the exception type is SomeError
or any
subclass of SomeError
.
try: dangerous_function() except SomeError: print("Error encountered!")
It may be helpful to refer to the class hierarchy for Python's built-in exception types. Here is a summary diagram of the most relevant exception classes:
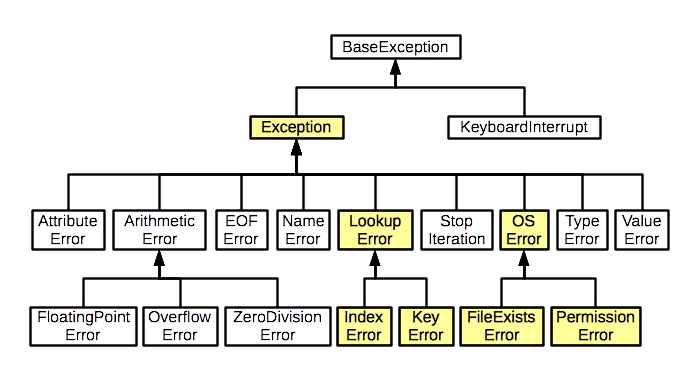
If the results don't match your predictions, take another look at the code to see if you can determine where you went wrong. If you can't make sense of the results, ask the instructor for help.
$ python3 word_writer.py hellos.txt hello 3
(Assuming thathellos.txt
does not exist.)$ python3 word_writer.py hellos.txt hello 3
(Assuming thathellos.txt
does exist.)$ python3 word_writer.py /hellos.txt hello 3
(Permission error.)$ python3 word_writer.py tmp.txt hello hello
Part 2: Improving The Script
The goal for this stage of the lab is to modify the script so that it responds appropriately to incorrect command line arguments. When bad arguments are provided, the script should print an informative error message followed by a usage string. Here are some examples:
$ python3 word_writer.py hellos.txt Incorrect number of arguments. Usage: word_writer.py FILE_NAME WORD COUNT $ python3 word_writer.py hellos.txt hello hello 'hello' cannot be converted to an integer. Usage: word_writer.py FILE_NAME WORD COUNT $ python3 word_writer.py hellos.txt hello 4 The file hellos.txt already exists. Usage: word_writer.py FILE_NAME WORD COUNT
As you modify the script, think about the best place to handle exceptions. There is little rhyme or reason to the original locations of the exception handlers: they were positioned to create interesting test cases for the previous exercise.
Part 3: Four-Letter Words
We must prevent this script from being used to generate files
containing four-letter words. Modify the print_words
function so
that it raises a FourLetterWordError
whenever the word
argument has exactly four letters. Your main
function should be
modified to catch this exception and print an appropriate error message:
$ python3 word_writer.py words.txt tree 100 The word 'tree' has four letters! Shame on you. Usage: word_writer.py FILE_NAME WORD COUNT
User defined exception types should extend the built-in
Exception
class. The following is perfectly acceptable:
class MyOwnError(Exception): pass
This declares a class that inherits all of its functionality from
Exception
, including the constructor.
Submission
This lab will not be graded so there is nothing to submit. Make sure you keep a copy of your code for future reference. If you would like to discuss your solution or any problems you encounter while working on this lab, please come to office hours or make an appointment.