Class Hierarchy Lab
Objectives
The goal of this lab is to gain experience with object-oriented programming in Python, and in particular with designing and implementing class hierarchies.
Introduction
In this lab, you will implement a class hierarchy of shape objects in Python, using
the
turtle
module to simplify the actual graphics implementation. All of
the information that you will need for this lab is in Chapter 2 of the class
textbook and the first lab.
Your class hierarchy should include the following classes:
- Shape (abstract base class)
- Circle
- Square
- Polygon
- Rectangle
Optional: If you have time, add the following classes:
- FilledPolygon
- FilledCircle
- Dot
Each of these represents a broad type or category of shapes with common
features. Instantiating these classes should result in objects that represent
particular concrete shapes that can be drawn using the turtle
module. We will be using the aggregated class hierarchy from the in-class
activity on Wednesday (9/3). Here is a simplified diagram of that hierarchy,
with a couple of minor modifications:
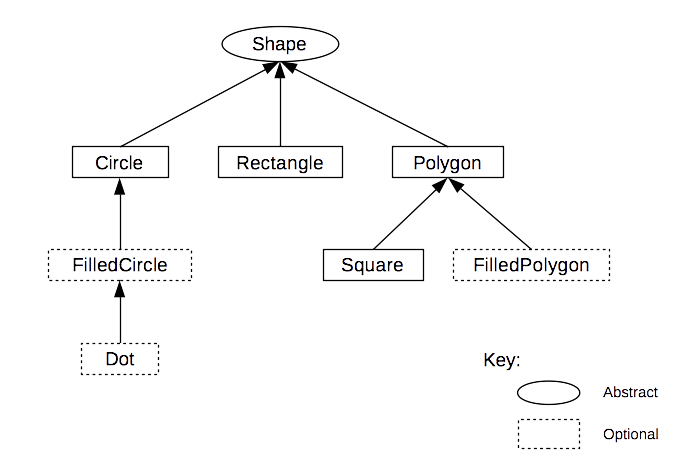
Note: In this lab, we will use the term "polygon" to mean "regular polygon."
Part 1 - Design
Draw a formal UML
class hierarchy based on the simplified diagram in the above section.
You will need to determine the member functions and attributes that should be
associated with each class. Include a draw()
method in the
Shape
abstract class. This draw()
method should take
no parameters and should be be implemented in subclasses as appropriate. Every
object should keep track of sufficient information to draw itself without
further input. You should look for opportunities to take advantage of
inheritance, minimizing duplicate code and member variables wherever possible.
Here is an example of what the UML diagram for the Polygon class should look like:
Polygon |
- x
|
+ draw() |
Part 2 - Implementation
Once you are satisfied with your class hierarchy, code up a Python
implementation using the turtle
module for graphics. All polygons
should be drawn starting at a given (x,y) point, moving to the right to draw the
initial edge and using left turns to draw remaining edges. All circles should be
drawn using the circle()
method in the turtle
module,
with the center of the circle located left of the given (x,y) point by the given
radius amount.
Include a main()
method that tests all of your
classes. Recall that we use the following snippet to import the turtle module:
from turtle import *
Also, recall the syntax for declaring abstract base classes in Python:
from abc import ABCMeta, abstractmethod class MyClass(metaclass=ABCMeta): @abstractmethod def do_something(): # subclasses pass # must implement
If your hierarchy is implemented correctly, you should be able to create a
list of shapes in main()
and then iterate over that list, calling
draw()
on every object regardless of what type of shape it is.
Submission
This lab will not be graded so there is nothing to submit. Make sure you keep a copy of your code for future reference. If you would like to discuss your solution or any problems you encounter while working on this lab, please come to office hours or make an appointment.