Lists and Strings Lab
Objectives
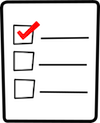
The goal of this lab is to get comfortable working with basic features of the Python programming language including Python lists, strings, and control flow constructs.
This lab is based on an in-class activity originally written by Nathan Sprague.
Introduction
In this lab, you will begin working with strings and lists in Python. Here are links to the pertinent sections of the Python documentation:
You may also be interested in the complete list of string methods.
Exercises
- Removing Letters
- Create a new Python code file to contain your code for this lab
(for example, you might title it "
strings.py
". Include the lab title, your name, and today's date in a comment at the top of the file. - Write a function with the following signature:
remove_letter(sentence, letter)
This function should take a string and a letter (as a single-character string) as arguments, returning a copy of that string with every instance of the indicated letter removed. For example,remove_letter("Hello there!", "e")
should return the string"Hllo thr!"
Hints:- Remember that a string is concatenation of individual
characters. Python allows you to iterate over the characters of
a string using the same syntax as a list iteration:
for ch in "Hello!": print(ch)
The above code snippet will print each character from the string"Hello!"
on a separate line. - Remember that the "+" symbol is used for string
concatenation. I can create the string
"Hi"
as follows:greeting = "" greeting = greeting + "H" greeting = greeting + "i"
Or equivalently:greeting = "" greeting += "H" greeting += "i"
- Remember that a string is concatenation of individual
characters. Python allows you to iterate over the characters of
a string using the same syntax as a list iteration:
- Add a
main()
function to your program and use it to testremove_letter
. Note that the following idiom is commonly used in Python and is required in this class as per the style guide:def main(): # your code goes here if __name__ == "__main__": main()
- Create a new Python code file to contain your code for this lab
(for example, you might title it "
- Find and Replace
- Write a function with the following signature:
replace_word(sentence, target, replacement)
This function takes three strings as arguments. The first string contains a sentence, the second contains a word to replace, and the third contains the replacement word. The return value should be a copy ofsentence
with every instance oftarget
replaced with an instance ofreplacement
. For example,replace_word("I am happy to meet you!", "happy", "angry")
should return the string"I am angry to meet you!"
. Don't worry about handling punctuation correctly. Punctuation marks may be considered part of the word that they follow. Try to write your function so that there is no trailing space in the returned sentence.
NOTE: Python strings have a built-inreplace
method that can accomplish this in a single line of code. Do not use it! The goal of this exercise is to practice implementing such functions. Solve the problem by iterating over the words in the string.
Hints:- Python strings have a
split
method that splits the string into a list of words. For example:greeting = "hello there" words = greeting.split()
After this snippet executions,words
will contain the list["hello", "there"]
. - You may wish to create a helper utility function that creates a string by joining all elements of a given list separated by spaces. Such a function may be useful in later exercises as well.
- Python strings have a
- Test your new function from
main()
. You should consider testing this function in conjunction withremove_letter
as follows:angry = replace_word("I am happy to meet you!", "happy", "angry") no_y = remove_letter(angry, "y") print(no_y)
The result should be"I am angr to meet ou!"
.
- Write a function with the following signature:
- Reversing a Sentence
- Write a function with the following signature:
reverse_sentence(sentence)
This function should take a string as an argument, and return a new string in which the order of the individual words has been reversed. Don't worry about separating and preserving punctuation; it's ok to treat it as if it was part of the word preceding it.
Hints:- There are at least two reasonable ways to solve this problem:
1) You could iterate forward through the existing words,
and append each subsequent word to the beginning of a new
string, or 2) You could iterate backward through the
existing words and append each subsequent word to the end
of a new string. Iterating backward can be accomplished either by
passing a negative third argument to the
range
function or by using negative list indexing (where -1 refers to the last element in the list, -2 to the second-to-last, etc.).
- There are at least two reasonable ways to solve this problem:
1) You could iterate forward through the existing words,
and append each subsequent word to the beginning of a new
string, or 2) You could iterate backward through the
existing words and append each subsequent word to the end
of a new string. Iterating backward can be accomplished either by
passing a negative third argument to the
- Test your new function from
main()
.
- Write a function with the following signature:
- String Formatting (optional)
- Write a function with the following signature:
format_str(description, values)
This function should return a reformatted version ofdescription
, where special identifier words like "$3
" have been replaced by the specified element fromvalues
. The special identifiers should always be a single word (no spaces) composed of a single dollar sign ("$") followed by the zero-based index of the desired value.
Your function should work regardless of whether the values are strings, integers, or floating-point numbers. Your function should also check to make sure the given index is within the bounds of the given list. If it is not, your function should insert the text "ERROR
".
Here are some examples:format_str("Hello $0", ["Mike"]) # result: "Hello Mike" format_str("The sum of $0 and $1 is $2", [3,5,8]) # result: "The sum of 3 and 5 is 8" format_str("The name is $1 -- $0 $1", ["James", "Bond"]) # result: "The name is Bond -- James Bond" format_str("Names: $0 $1 $2 $3", ["Mike", "Nathan"]) # result: "Names: Mike Nathan ERROR ERROR"
Hints:- To extract the index number from a special identifier, you may wish to use a technique called slicing. You can find information about it in the Python string documentation.
- Test your new function from
main()
.
- Write a function with the following signature:
Submission
This lab will not be graded so there is nothing to submit. Make sure you keep a copy of your code for future reference; you may also need it for a homework assignment. If you would like to discuss your solution or any problems you encounter while working on this lab, please come to office hours or post on Piazza.