Object-Oriented Design Lab
Categories:
3 minute read
Introduction
Frogger šø is a classic 2D sprite-based1 video game from the
1980’s. The goal for this exercise is to design an appropriate class
hierarchy for implementing this game in Java.
You may assume that you have access to a drawing library that provides
methods like the following:
StdDraw.drawImage(ImageObject image, double xPosition, double yPosition)
You may also assume that you have access to image files for each of the game entities and the game background.
Instructions
-
Carefully read the game specification below, underlining words that may correspond to classes in your finished hierarchy.
-
Brainstorm a class hierarchy that would allow you to implement the game logic with a minimum of code duplication. Look for opportunities to take advantage of polymorphism and dynamic binding. Make use of interfaces and abstract classes where appropriate.
-
Draw a “syntactically correct” UML diagram representing your design. You should include all public and protected methods, including constructors, as well as all instance variables.
Rubric
Your completed design will be evaluated according to the following rubric:
Item | Points |
---|---|
Design supports required functionality | 5 |
Correct UML syntax | 5 |
UML is visually well organized and neatly drawn. | 5 |
Appropriate names (Names are meaningful. Method names are verbs and instance variables and classes are nouns. Plural nouns are only used for collections.) | 5 |
Appropriate use of inheritance (Superclasses are used to prevent code duplication. Subclasses meaningfully extend the functionality of superclasses. Instance variables are not shadowed in subclasses.) | 5 |
Classes and methods are abstract where appropriate. | 5 |
Appropriate use of polymorphism | 5 |
Game Specification
The following screen-shot and description of game play are taken from the Frogger Wikipedia page :
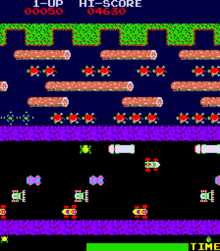
Frogger
The game starts with three, five, or seven frogs (lives), depending on the settings used by the operator. The player guides a frog which starts at the bottom of the screen, to his home in one of 5 slots at the top of the screen. The lower half of the screen contains a road with motor vehicles, which in various versions include cars, trucks, buses, dune buggies, bulldozers, vans, taxis, bicyclists, and/or motorcycles, speeding along it horizontally. The upper half of the screen consists of a river with logs, alligators, and turtles, all moving horizontally across the screen. The very top of the screen contains five “frog homes” which are the destinations for each frog. Every level is timed; the player must act quickly to finish each level before the time expires.
The only player control is the 4 direction joystick used to navigate the frog; each push in a direction causes the frog to hop once in that direction. On the bottom half of the screen, the player must successfully guide the frog between opposing lanes of trucks, cars, and other vehicles, to avoid becoming roadkill.
The middle of the screen, after the road, contains a median where the player must prepare to navigate the river.
By jumping on swiftly moving logs and the backs of turtles and alligators except the alligator jaws, the player can guide his or her frog safely to one of the empty lily pads. The player must avoid alligators sticking out at one of the five “frog homes”, snakes, and otters in the river, but may catch bugs or escort a frog friend for bonuses. When all five frogs are directed home, the game progresses to the next, level with an increased difficulty. After five levels, the game gets briefly easier yet again gets progressively harder to the next fifth level.
1 A sprite is a small image which may be moved.