Arrays Lab
Categories:
8 minute read
Background
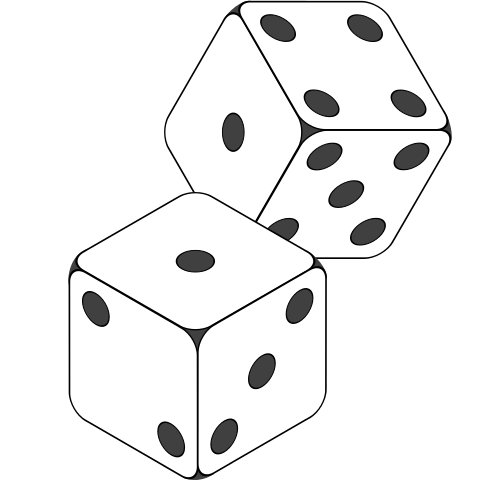
You will write today’s program from scratch. Create a new Java class named ArrayPlay
with a main
method. For each step below, you should add code to the program without replacing prior code. When you are finished, this program will provide you with a reference for working with arrays including initializing, printing, and comparing. This lab involves formatting String
s. If you haven’t completed the (Horstmann) Chapter 4 reading (especially 4.3.2), consider skimming it. You may also find the JDK documentation of the Formatter class (especially the Format String Syntax section) useful. Dr. Mayfield (et al.)’s ThinkJava2 available free online is also a handy supplemental resource (see e.g. its 3.5 Formatting Output , and consider learning this search engine operator since this version of the book doesn’t seem to provide a search mechanism).
🎲 Di(c)e
This lab assumes you have some level of familiarity with dice :
- specifically that the singular of “dice” is (unfortunately) “die” (😱💀 In my opinion, every language - natural and otherwise - has bugs 🪲, and this is one of many in English, don’t come at me, I also think it’s ridiculous. I think we get to blame this one on French ).
- dice (or a die) can be “thrown”, “rolled”, or “cast”, all meaning to drop it and let it land on a random face.
- in this lab, the “Die” we refer to is assumed to be a so-called D6 (as the gamers do) , meaning a die with 6 faces (or “sides”).
🛑🧐 Stop and check:
Ensure that:
- the
ArrayPlay
class you created is spelled correctly (including upper- vs. lowercase) - the
ArrayPlay
class is in the default package (i.e. nopackage
statement in the source code, and the Eclipse Package Explorer shows the class in the(default package)
)
Objectives
- Use an array to store a sequence of values.
- Display the contents of an array with a loop.
- Practice manipulating various types of arrays.
- Write methods using array parameters.
Key Terms
- Array Initializer
- list of values used to instantiate and initialize an array in one step (see more on
Array Creation Expressions vs. Array Initializers, if you're curiousactually those both go to higher dimensional arrays which is out of scope for this activity. Probably skimming ThinkJava2's Creating Arrays is a sufficient quick reference) array.length
- read-only attribute of an array that stores the number of elements
- subscript (also "index")
- integer value in brackets [ ] that specifies an element of an array
ArrayIndexOutOfBoundsException
- error raised when trying to access
a[i]
wherei < 0
ori >= a.length
Part 1: Sequentially accessing an array
Whenever you are asked to do something to your array and then print it, you should finish the update step first and then have a separate loop to do the printing.
- In the
main
method, create a single array of integers that is6
elements long. - Initialize each of the array elements to the value
-1
using a loop of your choice. - In another loop, print each element of the array with messages that look like:
array[0] = -1 array[1] = -1 ...
- Add code to change the value of each element of the array to its subscript. For example,
array[3]
should hold the value3
. - Print the new contents of the array with the same message format as step 3. (You may copy and paste the code from step 3. Your program should now print both versions of the array.)
- add code to re-initialize your array contents to all zeros.
- Print the string
Array Play
(on its own line) to conclude Part 1.
Part 2: Randomly accessing an array
- Download Die.class for use in this Part and later. Assuming you created the Prerequisite File Hierarchy, you should then:
- Create a new directory (call it something about this activity, e.g.
lab04
) in theprovided
directory. - move the
Die.class
file you downloaded into the newly directory. - In Eclipse, right-click on the the current Java Project you’re using, and choose
Properties...
(last entry in thecontext menu
). - in the left pane, select
Java Build Path
. - in the right pane, select the tab, labeled
Libraries
. - click the
Classpath
item in the center pane. - click the button no the far right, labeled
Add External Class Folder...
. - navigate to the
provided
directory and select thelab04
directory. - Click
Apply and Close
.
- Create a new directory (call it something about this activity, e.g.
- Create a new Die object.
- (Refer to the Die javadoc for the documentation for the class
Die
.) - If the compiler insists
Die cannot be resolved to a type
, it’s likely you made an error in the preceding steps to add the class folder, or need to double-check the 🛑🧐 Stop and check after the background section above.
- (Refer to the Die javadoc for the documentation for the class
- Create a loop that will iterate at least 100 times. In the loop body:
- Roll the die. (Don’t create a new object, just roll it again.)
- Based on the result of the roll, increment the corresponding cell of your array. The value of each array element should be the number of times that roll is made.
- After the loop finishes, print the results of the simulation. For example, if the array holds
{20, 17, 19, 15, 12, 17}
you would output (notice the empty line between each message):1 was rolled 20 times. 2 was rolled 17 times. ...
Part 3: Working with multiple arrays
For this exercise, you will need two arrays of double values (I think I’ll name mine, minogue
and oLeary
).
- Use an array initializer { } to assign one of the arrays to
10
double
values starting at0.0
with each element1.0
more than the previous (ending with the last element being set to9.0
). - Instantiate the other array to store 10 elements, but do not initialize the contents yet.
- Print the contents of both arrays side by side on your screen, but first label this output by printing
Before
.- format the output as follows:
Before 0 A1 = 0.0 A2 = 0.0 1 A1 = 1.0 A2 = 0.0 ...
- Copy the contents of the first array into the second, then print their contents again. Label this output
After
. - Finally, change the element at index
0
of the first array to99.0
and the element at index5
of the second array to99.0
. - Again, print the contents of the two arrays side by side. Label this output
After Change
. - Check that the contents of the two arrays are indeed different. If not, make the appropriate corrections.
This is the expected output file for ArrayPlay
: ArrayPlay.exp.
Part 4: Refactoring
In the lab so far, you were required to print all of the contents of an array several different times. Perhaps (as the instructions permitted) you did this by copying and pasting the same code several times. This is a bad practice. Instead, you should write a method that prints the contents of an array. This method should take the array as a parameter and print the contents of the array. You should then call this method from the appropriate places in your code.
🤔Why?
- Why is it a bad practice to copy and paste?
- Why is it better to write a method?
- What are the advantages and disadvantages of copy/paste vs. creating and invoking a method?
- Consider saving a copy of
ArrayPlay.java
as you have it now somewhere else, and after completing and submitting this lab, change the behavior of the class up to this point to have a different format of message when printing. Compare that to the code you submit to Gradescope for this activity.
+printArray(a: int[])
Write a (static
and void
) method named printArray
that prints the contents of an array. The method should take the array as a parameter and print the contents of the array following the message format from Part 1. If the array is null
, it should print the String null
. If the array is empty, it should not print anything. You should then replace the code in your main
method that prints the contents of the array with a call to this method.
+formMessage(a: int[], index: int, format: String): String
Write a static
method named formMessage
that defines 3 formal parameters:
a
is an array of integers.index
is an integer that is the index of the array.format
is aString
that is a format string for the message. and from these parameters, returns aString
that is the message for the provided array’s element at positionindex
, with the givenformat
. For example, ifa
holds{20, 17, 19, 15, 12, 17}
,index
is3
, andformat
isarray[%d] = %d\n
, the message would be:array[3] = 15\n
. You should then replace the code in yourprintArray
method that constructs theString
to print with a call to this method. Note:- you should not print anything in this method.
- if the provided array is null, you should return the String
null
. - if the provided index is out of bounds, you should return the String
index out of bounds
.
+printArray(a: double[], b: double[])
Overload the printArray
method to take two arrays of doubles as parameters. The method should print the contents of the two arrays side by side, with the first array’s elements on the left and the second array’s elements on the right. The method should print the contents of the arrays following the message format from Part 3. If either array is null
, it should print the String null
. If either array is empty, or if the arrays are not the same length as each other, it should not print anything. You should then replace the code in your main
method that prints the contents of the arrays with a call to this method.
Submitting
Submit your final ArrayPlay.java
file to Gradescope.
Acknowledgements 🙏
This lab is with thanks to at least Alvin Chao (, but likely also Nathan Sprague and David Bernstein ).