HW5: Rave'n
9 minute read
Homework 5
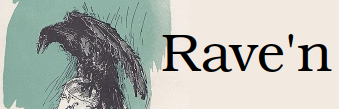
🛑 To Know Before You Start
As discussed below, on this assignment your grade will be reduced by 5 points for each submission after the 10th submission. Hence, you should be confident that your code is correct before you submit the first time.Learning Objectives
This homework assignment is designed to help you learn about reference types, references, and the difference between aliases and deep copies. It will also, because it is a little longer than previous homework assignments, convince you to start working on assignments early (which will be necessary for the programming assignments to come). Finally, it will, hopefully, teach you the importance of testing your code before you submit it (even though testing is not required).
Overview
As you know, students in the Computer Science Department are famous for their wild parties. As you also know, they sometimes don’t spend enough time planning (whether it be for assignments or parties). As a result, they sometimes work on assignments at the last second, and sometimes don’t have enough food and drink for the number of people that attend their parties. The Department Head has decided that she would like to see fewer failed parties, so has contracted with you to create a party planning tool named Rave’n. (She’ll try to tackle the failed assignment problem later.)
The Classes to be Written
You must write three classes named Item
, Order
, and Invoice
(all
of which must be in the deault package) that are summarized on the
following UML class diagram.
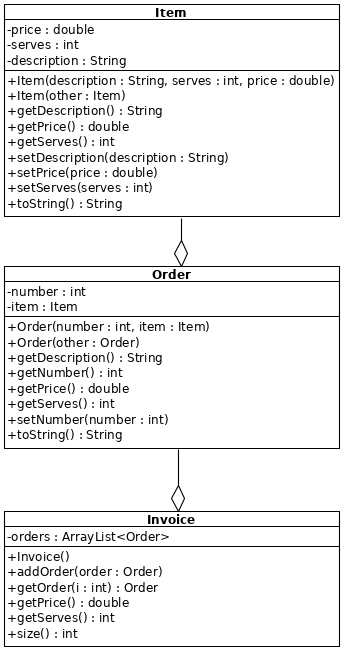
As shown in this diagram, an Order
consists of an Item
and a
number, and an Invoice
consists of 0 or more Order
objects. For
example, one Item
that is commonly found at CS parties is pizza,
which might have a description
attribute of "Pizza"
, a serves
attribute of 3
people, and a price
attribute of 8.99
. A typical
CS party might have 600 people in attendance, so the planners might
place an Order
for this Item
with a number
attribute of 200
. A
typical CS party might also serve several other things all of which
would be incorporated in an Invoice
.
Detailed Design Specifications
In addition to the specifications contained in the UML class diagram, your implementation must conform to the following specifications.
The Item
Class
The description
attribute must be an alias of the parameter that is
passed to the explicit value constructor, and the copy constructor
must invoke the explicit value constructor.
The toString()
method must return a String
representation of the
description
, serves
, and price
attributes that is formated using
"%s for %d at $%5.2f"
.
The Order
Class
The explicit value constructor must make a deep copy of the item
parameter, and the copy constuctor must invoke the explicit value
constructor.
The getServes()
method and the getPrice()
method must return the
corresponding values in the item
attribute multiplied by the
number
attribute. For example, suppose an Item
of pizza serves 3
at a cost of $8.99 and the number
in the Order
is 7
. Then the
getServes()
method must return 21
and the getPrice()
method must
return 62.93
. The getDescription()
method must return the corresponding value from the item
attribute.
The toString()
method must return the number
attribute and the
value returned by the item
attributes toString()
method formatted
using "%d orders of %s"
.
The Invoice
Class
The constructor must initialize the orders
attribute.
The addOrder()
method must add an alias of the parameter named
order
to the attribute named orders
.
The getOrder(int)
method must return null
when the value of the
parameter is out of bounds. Otherwise, it must return a reference to
the appropriate element in the orders
attribute.
The getPrice()
method must return the total price of all Order
objects in the orders
attribute. For example, suppose the Invoice
contains $62.93 worth of pizza, $10.72 worth of soda, and $41.64 worth
of chips. Then, this method must return 115.29.
The getServes()
method must return 0 when there are no Order
objects in the orders
attribute. Otherwise, it must return the
minimum value of all of the serves
attributes associated with the
Order
objects in the orders
attribute. For example, suppose the
Invoice
contains enough pizza to serve 21, enough soda to serve 20,
and enough chips to serve 24. Then, this method must return 20
.
The size()
method must return the number of elements in the orders
attribute.
Testing
You may test your code in either or both of the following two ways. How you test your code is up to you. But, you should be aware of the fact that Gradescope will not provide much help.
Unit Testing Using JUnit
You can test your code using JUnit. (You should not use the Test
class from the earlier assignments any longer, especially since you
will be required to use JUnit in the future.)
System Testing Using a Graphical User Interface
You can test the entire system using a graphical user interface (GUI)
that was written by the Department. It is available as a .jar
file.
Help incoroprating it into your project is available on the CS Wiki .
To use it you will need to create (and execute) the following main class.
import java.lang.reflect.InvocationTargetException;
import javax.swing.SwingUtilities;
/**
* This is the main class for Rave'n.
*
* @author Computer Science Department, James Madison University
* @version 1
*/
public class Raven {
/**
* The entry point of the application.
*
* @param args The command-line arguments (which are not used).
* @throws InterruptedException
* @throws InvocationTargetException
*/
public static void main(String[] args)
throws InterruptedException, InvocationTargetException {
RavenWindow window;
window = new RavenWindow();
SwingUtilities.invokeAndWait(window);
}
}
The RavenWindow
object that is constructed in the main()
method
will make use of your Item
, Order
, and Invoice
classes.
Submission
You must submit (using Gradescope):
- Your implementation of the
Item
,Order
, andInvoice
classes.
Do not submit any unit tests.
Your grade will be reduced by 5 points for each submission after the 10th submission. So, you should try to ensure that your code is correct before you submit it the first time.
Grading
Your code will first be graded by Gradescope and then by the Professor. The grade you receive from Gradescope is the maximum grade that you can receive on the assignment
Gradescope Grading
Your code must compile (in Gradescope, this will be indicated in the section on “Does your code compile?”) and all class names and method signatures must comply with the specifications (in Gradescope, this will be indicated in the section on “Do your class names, method signatures, etc. comply with the specifications?”) for you to receive any points on this assignment. Gradescope will then grade your submission as follows:
Criterion | Points | Details |
---|---|---|
Conformance to the Style Guide | 0 points | (Success Required) |
Correctness | 100 points | (Partial Credit Possible) |
As discussed above, your grade will be reduced by 5 points for each submission after the 10th submission. Gradescope will provide you with hints, but may not completely identify the defects in your submission.
Manual Grading
After the due date, the Professor may manually review your code. At this time, points may be deducted for inelegant code, inappropriate variable names, bad comments, etc.
Recommended Process
Since nobody will be looking over your shoulder, you can use any process that you would like to use. However, it is strongly recommended that you use the process described here.
Get Started
- Read and understand the entire assignment.
- Create a project for this assignment named
hw5
. (Remember, do not create a module and create separate folders for source and class files). - Activate Checkstyle for this assignment.
Stub-Out the Classes
- Stub-out the
Item
class. - Stub-out the
Order
class. - Stub-out the
Invoice
class. - Add the “javadoc” comments to the three classes class.
- Check the style of the three classes and make any necessary corrections.
Implement and Test (if Desired) the Classes
- Implement and test everything in the
Item
class except the copy constructor. - Implement and test the copy constructor in the
Item
class. - Implement and test the explicit value contstructor and simple getters in the
Order
class. - Implement and test the
toString()
method in theOrder
class. - Implement and gest the
getServes()
method in theOrder
class. - Implement and gest the
getPrice()
method in theOrder
class. - Implement and test the copy constructor in the
Order
class. - Implement and test the constructor,
addOrder()
,getOrder()
, andsize()
methods in theInvoice
class. - Implement and test the
getPrice()
method in theInvoice
class. - Implement and test the
getServes()
method in theInvoice
class.
Conduct System Tests (if Desired)
- Download
hw5.jar
to a directory outside of your Eclipse workspace (e.g., thedownloads
directory for this course). - Add
hw5.jar
to the project (using the steps described on the CS Department’s wiki)[https://wiki.cs.jmu.edu/student/eclipse/help#using_third-party_classjar_files]. - Execute the
Raven
class. - Add a few orders.
- Ensure that the information displayed is correct.
- Change the number of items in one order.
- Ensure that the information displayed is correct.
- Change the number of items in another order.
- Ensure that the information displayed is correct.
The following screenshot shows an example of how the GUI might be used.
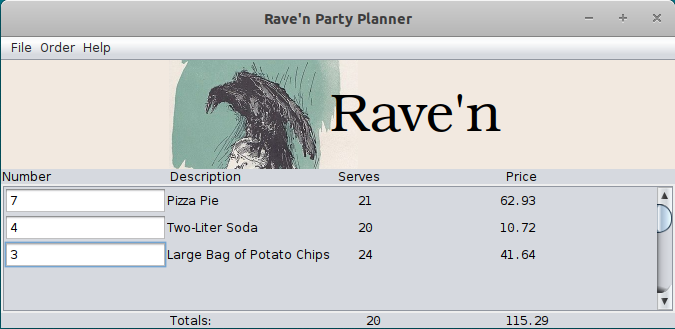
In this example, the pizza serves 3 and costs $8.99, the bottle of soda serves 5 and costs $2.68, and the bag of chips serves 8 and costs $13.88.
Requesting Help About Failed Official Tests
When requesting help about failed official tests, whether from a learning assistant or a Professor,
you must present the tests that you have run that you believe address the issue identified in
the failed test. For example, if Gradescope indicates that your getServes()
method in the Invoice
class failed a test, you must provide the unit tests or system tests that you wrote to verify
the correctness of that method. Of course, you must not post your tests on Piazza.
Questions to Think About
You don’t have to submit your answers to these questions, but you should try to answer them because they will help you determine whether or not you understand some of the important concepts covered in this assignment.
- What does the word “deep” really mean when used in the context of a “deep copy”? Can there be different levels of depth?
- Why is it sometimes appropriate to use an alias and sometimes appropriate to use a deep copy?
- Why did we not require you to submit unit tests for this assignment even though we think that they are necessary?